Understanding “subprocess-exited-with-error” in Python
When working with Python and external processes, the subprocess module is a powerful tool that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes.
However, encountering the error message “subprocess-exited-with-error” can be puzzling for many developers. In this article, we will explore what this error means, common causes, and how to handle it effectively.
What is subprocess-exited-with-error?
The “subprocess-exited-with-error” message typically appears when a subprocess launched by Python exits with a non-zero return code.
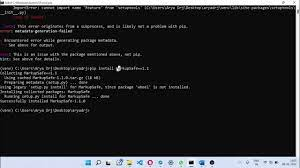
In other words, the external command or process encountered an error during its execution. The subprocess module captures this error by checking the return code of the subprocess and raises a CalledProcessError exception when the return code is non-zero.
Here’s a simple example demonstrating how this error might occur:
try:
subprocess.run([‘ls’, ‘nonexistent_directory’])
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
In this example, the ls command is used to list the contents of a directory that doesn’t exist. The subprocess.run function raises a CalledProcessError because the ls command exits with a non-zero return code when it fails to find the specified directory.
Common Causes of subprocess-exited-with-error:
1. Command Execution Failure:
The most common cause is that the command or process executed by subprocess.run encountered an error during its execution.
It could be due to invalid command-line arguments, missing dependencies, or issues with the external program itself.
2. Incorrect Command or Arguments:
Ensure that the command and its arguments passed to subprocess.run are correct. Typos or incorrect parameters can lead to command failures.
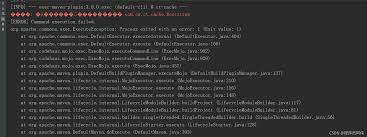
3. Environment Issues:
The external process might depend on certain environment variables or configurations that are not set correctly when invoked from Python.
4. Permissions:
If the external command requires certain permissions that the Python process lacks, it may result in an error.
5. Handling subprocess-exited-with-error:
When encountering this error, it’s crucial to handle it gracefully. Here are some approaches:
6. Capture Output for Debugging:
Use the subprocess.CalledProcessError.output attribute to access the output of the failed command. This can provide valuable information for debugging.
SARGARPGIO: YOUR GATEWAY TO REVOLUTIONARY AI TEXT ROLE-PLAYING ADVENTURES
try:
result = subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
print(f”Output: {e.output}”)
7. Logging:
Integrate logging to record details about the error, the command executed, and any other relevant information.
logging.basicConfig(level=logging.INFO)
try:
subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
logging.error(f”Error: {e}”)
logging.error(f”Output: {e.output}”)
8. Handle Specific Errors:
Catch specific exceptions related to the subprocess error, allowing for more granular error handling.
try:
subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True)
except FileNotFoundError:
print(“The specified command was not found.”)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
Understanding Return Codes:
The return code of a subprocess is a numerical value that indicates the exit status of the executed command. A return code of 0 usually signifies successful execution, while a non-zero value indicates an error.
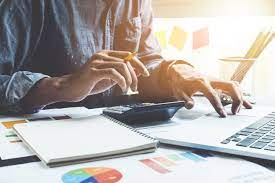
Understanding the specific return codes associated with a subprocess can offer valuable insights into the nature of the encountered issue. Consult the documentation of the external command or program to interpret the meaning of different return codes, helping you pinpoint the root cause of the error more accurately.
try:
result = subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
print(f”Return Code: {e.returncode}”)
By examining the return code, you can implement conditional logic in your Python script to take appropriate actions based on the specific error scenario.
Handling Standard Error Output:
In addition to capturing standard output, it’s essential to capture and analyze standard error (stderr) output. Standard error is where error messages and diagnostic information are typically written by commands. By capturing stderr, you can obtain more detailed information about what went wrong during the subprocess execution.
SARGARPGIO: YOUR GATEWAY TO REVOLUTIONARY AI TEXT ROLE-PLAYING ADVENTURES
try:
result = subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True, stderr=subprocess.PIPE)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
print(f”Standard Error: {e.stderr}”)
Analyzing the stderr output can provide crucial details for troubleshooting and resolving the issue.
Timeouts and Subprocess Deadlocks:
When working with subprocesses, it’s important to consider the possibility of timeouts and deadlocks. A subprocess might hang indefinitely, leading to a deadlock situation. To mitigate this, you can set a timeout for the subprocess using the timeout parameter in subprocess.run. This ensures that if the subprocess takes longer than the specified duration, it will be terminated.
try:
result = subprocess.run([‘ls’, ‘nonexistent_directory’], check=True, capture_output=True, text=True, timeout=5)
except subprocess.TimeoutExpired as e:
print(f”Error: {e}”)
print(“The subprocess timed out.”)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
Setting appropriate timeouts helps prevent your Python script from getting stuck indefinitely, especially when dealing with external processes that may have unpredictable execution times.
Cross-Platform Considerations:
Keep in mind that subprocess behavior may vary across different operating systems (OS). Commands and their options can be OS-specific, and certain features may not be available on all platforms. When developing Python scripts that involve subprocesses, account for these platform differences and ensure that your code remains robust and functional across various operating systems.
THE TIMBIGUER PHENOMENON: UNRAVELING THE ENIGMA OF ITS IMPACT
command = [‘ls’, ‘nonexistent_directory’]
if platform.system() == ‘Windows’:
# Adjust the command or handle Windows-specific cases
command = [‘dir’, ‘nonexistent_directory’]
try:
result = subprocess.run(command, check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
print(f”Error: {e}”)
Conclusion:
In conclusion, the “subprocess-exited-with-error” message signals that an external process executed by Python has encountered an issue. Understanding the causes and implementing effective error handling strategies will aid in debugging and improving the robustness of your Python scripts or applications.
You May Also Like
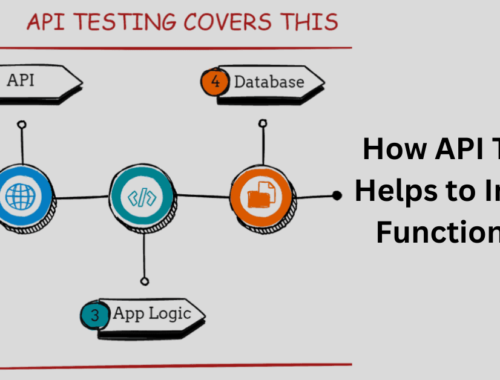
How API Testing Helps to Improve Functionality?
September 14, 2023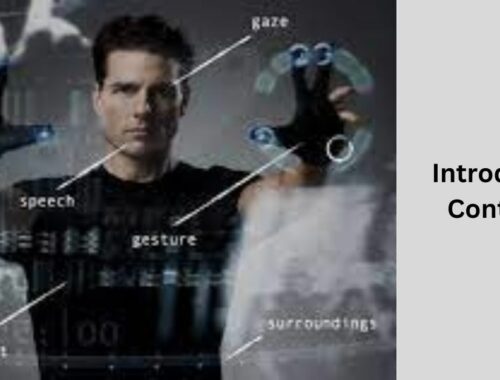
Introducing Contexto: The Wordle Spin-Off Taking Social Media by Storm
March 1, 2024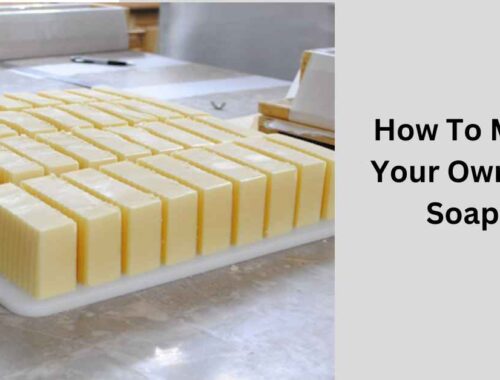